A constructor is a special method in Python that is called when you create a new instance of a class. The constructor is used to set up the initial state of the object. It is also used to allocate memory for the object. The constructor is called when you create an object using the keyword new. The constructor is not called when you create an object using the keyword class.
What is a constructor in Python and what does it do?
A constructor is a special method in Python that is used to set up the initial state of an object. It is also used to allocate memory for the object. The constructor is called when you create an object using the keyword new. The constructor is not called when you create an object using the keyword class. The constructor is used to set up the initial state of an object. This includes setting any instance variables that the object has. The constructor is also responsible for allocating memory for the object.
When should you use a constructor in Python?
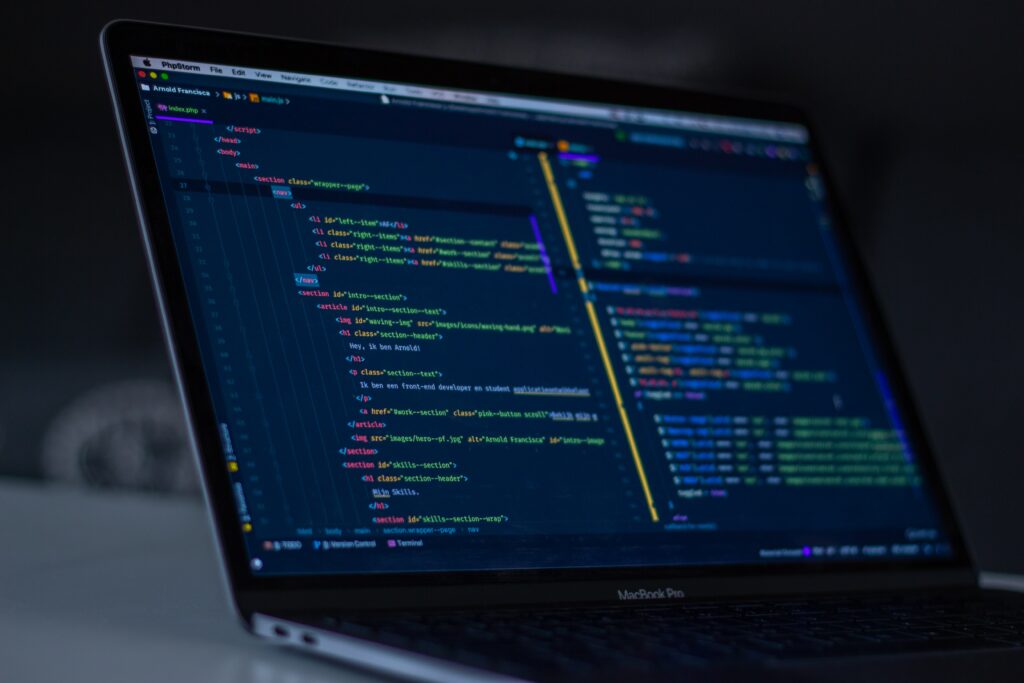
You should use a constructor when you want to set up the initial state of an object. You should also use a constructor when you want to allocate memory for an object. Constructors are typically used when you create an object using the keyword new. However, they can also be used when you create an object using the keyword class.
What is a constructor in python
The constructor is called when you create an object using the keyword new. The constructor is not called when you create an object using the keyword class. The constructor has two parameters, self and args. The self parameter is used to reference the object itself. The args parameter is used to pass arguments to the constructor.
When you create a new instance of a class, the __init__() method is called. The __init__() method is used to initialize the state of the object. It is also used to allocate memory for the object. The __init__() method has two parameters, self, and args. The self parameter is used to reference the object itself. The args parameter is used to pass arguments to the __init__() method.
What are the benefits of using a constructor in Python?
Some benefits of using a constructor in Python include:
• You can set up the initial state of an object.
• You can allocate memory for an object.
• Constructors are typically used when you create an object using the keyword new.
• However, they can also be used when you create an object using the keyword class. This can be helpful if you want to create multiple objects that have the same initial state.
• Constructors can help to make your code more readable and maintainable.
How do you create a constructor for your own classes in Python?
To create a constructor for your own classes in Python, you need to define a special method This method will be called when you create a new instance of the class. The method can be used to set up the initial state of the object. It can also be used to allocate memory for the object. The method has two parameters, self, and args. The self parameter is used to reference the object itself. The args parameter is used to pass arguments to the method. You can add additional parameters to the method if you want. However, self and args must always be the first two parameters.
How can you use constructors to improve the usability of your Python codebase?
Constructors can help to make your code more readable and maintainable. They can also help you to avoid duplication in your code. Additionally, constructors can be used to set up the initial state of an object. Finally, constructors can be used to allocate memory for an object. All of these things can help to improve the usability of your Python codebase.